How I made my Dell R710 quiet π WITHOUT hardware mods
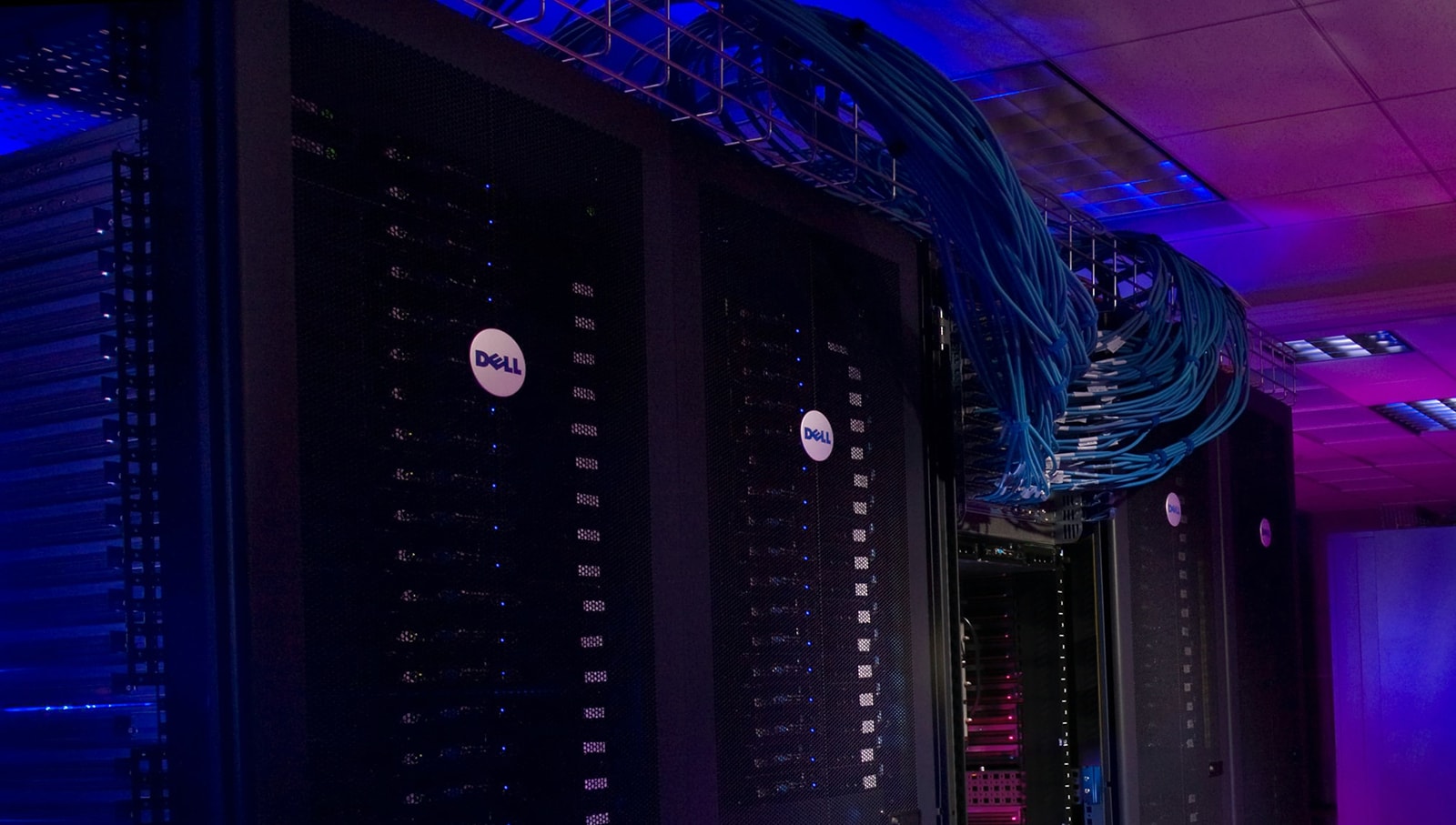
I created a BASH script for my Dell R710 to check the temperature, and if it's higher than XX (27 degrees C in my case) it sends a raw command to restore automatic fan control.
#!/usr/bin/env bash
# ----------------------------------------------------------------------------------
# Script for setting manual fan speed to 2160 RPM (on my R710)
#
# Requires:
# ipmitool β apt-get install ipmitool
# slacktee.sh β https://github.com/course-hero/slacktee
# ----------------------------------------------------------------------------------
# IPMI SETTINGS:
# Modify to suit your needs.
# DEFAULT IP: 192.168.0.120
IPMIHOST=10.0.100.20
IPMIUSER=root
IPMIPW=calvin
IPMIEK=0000000000000000000000000000000000000000
printf "Activating manual fan speeds! (2160 RPM)" | systemd-cat -t R710-IPMI-TEMP
echo "Activating manual fan speeds! (2160 RPM)" | slacktee.sh -t "R710-IPMI-TEMP [$(hostname)]"
ipmitool -I lanplus -H $IPMIHOST -U $IPMIUSER -P $IPMIPW -y $IPMIEK raw 0x30 0x30 0x01 0x00
ipmitool -I lanplus -H $IPMIHOST -U $IPMIUSER -P $IPMIPW -y $IPMIEK raw 0x30 0x30 0x02 0xff 0x09
#!/bin/bash
# ----------------------------------------------------------------------------------
# Script for checking the temperature reported by the ambient temperature sensor,
# and if deemed too high send the raw IPMI command to enable dynamic fan control.
#
# Requires:
# ipmitool β apt-get install ipmitool
# slacktee.sh β https://github.com/course-hero/slacktee
# ----------------------------------------------------------------------------------
# IPMI SETTINGS:
# Modify to suit your needs.
# DEFAULT IP: 192.168.0.120
IPMIHOST=10.0.100.20
IPMIUSER=root
IPMIPW=calvin
IPMIEK=0000000000000000000000000000000000000000
# TEMPERATURE
# Change this to the temperature in celcius you are comfortable with.
# If the temperature goes above the set degrees it will send raw IPMI command to enable dynamic fan control
MAXTEMP=27
# This variable sends a IPMI command to get the temperature, and outputs it as two digits.
# Do not edit unless you know what you do.
TEMP=$(ipmitool -I lanplus -H $IPMIHOST -U $IPMIUSER -P $IPMIPW -y $IPMIEK sdr type temperature |grep Ambient |grep degrees |grep -Po '\d{2}' | tail -1)
if [[ $TEMP > $MAXTEMP ]];
then
printf "Warning: Temperature is too high! Activating dynamic fan control! ($TEMP C)" | systemd-cat -t R710-IPMI-TEMP
echo "Warning: Temperature is too high! Activating dynamic fan control! ($TEMP C)" | /usr/bin/slacktee.sh -t "R710-IPMI-TEMP [$(hostname)]"
ipmitool -I lanplus -H $IPMIHOST -U $IPMIUSER -P $IPMIPW -y $IPMIEK raw 0x30 0x30 0x01 0x01
else
# healthchecks.io
curl -fsS --retry 3 https://hchk.io/XXX >/dev/null 2>&1
printf "Temperature is OK ($TEMP C)" | systemd-cat -t R710-IPMI-TEMP
echo "Temperature is OK ($TEMP C)"
fi
I’m running this on an Ubuntu VM on ESXi (on the R710 box), but it should be able to run as long as you have ipmitools. It could be you need to modify the logging, to make it work with whatever your system use.
I run the script via CRON every 5 minutes from my Ubuntu Server VM running on ESXi.
*/5 * * * * /bin/bash /path/to/script/R710-IPMITemp.sh > /dev/null 2>&1
Notice that I use healthchecks.io in the script to notify if the temp goes to high (it would also trigger if the internet goes down for some reason). Remember to get your own check URL if you want it, or else just remove the curl command.
I’m also currently testing out slacktee.sh to get notifications in my slack channel.
Howto: Setting the fan speed of the Dell R610/R710
- Enable IPMI in iDrac
- Install ipmitool on linux, win or mac os
- Run the following command to issue IPMI commands:
ipmitool -I lanplus -H <iDracip> -U root -P <rootpw> <command>
Enable manual/static fan speed:
raw 0x30 0x30 0x01 0x00
Set fan speed:
(Use i.e http://www.hexadecimaldictionary.com/hexadecimal/0x14/ to calculate speed from decimal to hex)
3000 RPM: raw 0x30 0x30 0x02 0xff 0x10
2160 RPM: raw 0x30 0x30 0x02 0xff 0x0a
1560 RPM: raw 0x30 0x30 0x02 0xff 0x09
Note: The RPM may differ from model to model
Disable / Return to automatic fan control:
raw 0x30 0x30 0x01 0x01
Other: List all output from IPMI
sdr elist all
Example of a command:
ipmitool -I lanplus -H 192.168.0.120 -U root -P calvin raw 0x30 0x30 0x02 0xff 0x10
Disclaimer
I’m by no means good at IPMI, BASH scripting or regex, etc. but it seems to work fine for me.
TLDR; I take NO responsibility if you mess up anything.
All of this was inspired by this Reddit post by /u/whitekidney